F distribution
F distribution¶
Letâs investigate how shape of the F distribution curve look likes.
# import required libraries
import numpy as np
import matplotlib.pyplot as plt
import scipy.stats as stats
from IPython.display import display
Letâs now investigate how shape of the F distribution curve changes with respect to degrees of freedom (\(\nu_1\) and \(\nu_2\)). We will define a function first to simplify our calculations.
# define a Python function with three parameters
def f_dist_curve(dof1,dof2,ltype, col):
fig, ax = plt.subplots(figsize = (6, 6))
#use zip for parallel iteration
for d1, d2, l, cl in zip(dof1, dof2, ltype, col):
#generate 10000 random variables for given degrees of freedom
x = stats.f.rvs(dfn = d1, dfd = d2, size = 10000)
x1 = np.sort(x)
plt.plot(x1, stats.f.pdf(x1, dfn = d1, dfd = d2),
ls = l, c = cl, label = r'$\nu_1=%.1f,\nu_2=%.1f$' % (d1,d2))
plt.xlim(0, 5)
plt.ylim(0, 0.8)
plt.xlabel('$x$')
plt.ylabel(r'$f(x|\nu_1,\nu_2)$')
plt.title('F distribution curve')
plt.legend(loc='upper right')
plt.show()
Now play with the function to see the changes on the normal distribution curve.
## change the following values as you wish
## define the input arguments (values of the parameters)
dof1_values = [4, 4, 4, 4]
dof2_values = [4, 10, 20, 30]
linestyles = ['-', '--', '-.', ':']
colors = ['blue', 'green','red','black']
f_curve = f_dist_curve(dof1 = dof1_values, dof2 = dof2_values, ltype = linestyles, col = colors)
display(f_curve)
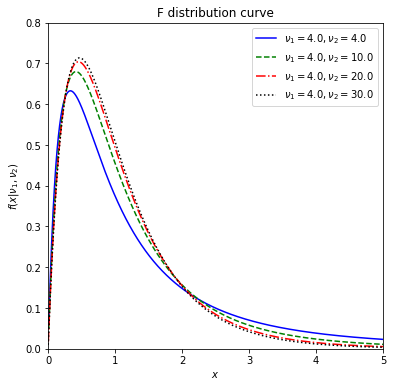
None
Calculate probabilities associated with chi-square distribution with âstats.f.cdf(x, dfn, dfd)â.